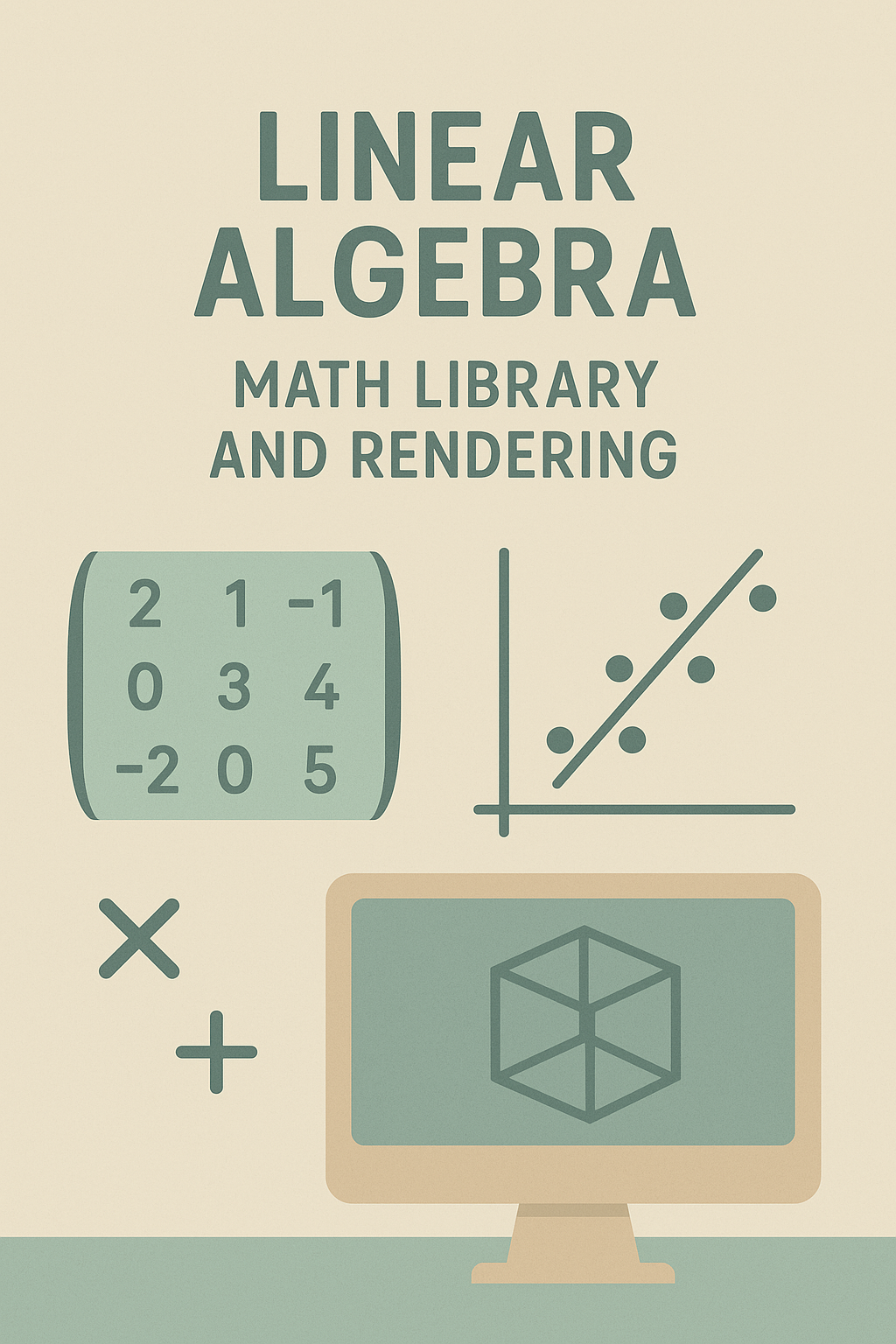
Low-Level Graphics Rendering with C
A custom C library implementing linear algebra fundamentals to render 2D/3D graphics directly to the Linux framebuffer, bypassing high-level graphics APIs.
C Linear Algebra Engine for Direct Framebuffer Rendering
Project Overview
This project implements a lightweight graphics rendering engine entirely in C. It combines a custom linear algebra library (handling vectors and matrices) with direct manipulation of the Linux framebuffer (/dev/fb*
). The primary goal is to render simple 2D and potentially 3D scenes without relying on external graphics libraries like OpenGL, Vulkan, SDL, or X11/Wayland, offering a deep dive into the fundamentals of graphics programming.
The engine provides the building blocks for drawing primitives and transforming objects, demonstrating core concepts from matrix transformations to pixel plotting on raw display hardware.
Purpose
Why Direct Framebuffer Rendering?
Modern graphics development often involves high-level APIs that abstract away the underlying hardware interactions. While powerful, these abstractions can obscure the fundamental principles of how images are actually drawn to a screen. This project aims to:
- Demystify Graphics: Gain a practical understanding of the graphics pipeline, from mathematical transformations to final pixel color calculations.
- Master C Fundamentals: Apply C programming concepts like memory management, pointers, and low-level I/O in a challenging and visual domain.
- Explore Linux Internals: Interact directly with a core Linux device interface (the framebuffer).
- Build from Scratch: Appreciate the complexity and design choices involved in creating even basic graphics capabilities.
- Minimalism: Create a rendering system with minimal external dependencies, suitable for embedded systems or environments without standard graphics stacks.
Key Features
(Note: Tailor this section based on what you actually implemented)
1. Custom Linear Algebra Library
- Vector Operations: Implementation of 2D/3D/4D vector types and operations (addition, subtraction, dot product, cross product, magnitude, normalization).
- Matrix Operations: Implementation of 3x3/4x4 matrix types and operations (multiplication, potentially identity, transpose, inverse).
- Transformation Matrices: Functions to generate standard graphics transformation matrices (translation, rotation, scaling, perspective/orthographic projection).
2. Direct Framebuffer Interface
- Device Interaction: Code to open, query (resolution, color depth, stride), and memory-map the Linux framebuffer device (
/dev/fb*
). - Pixel Plotting: A core function (
draw_pixel(x, y, color)
) capable of writing color data to the correct memory location in the mapped framebuffer, handling different pixel formats (e.g., RGB565, RGB8888).
3. Rendering Primitives
- Line Drawing: Implementation of an efficient line drawing algorithm (e.g., Bresenham’s line algorithm).
- (Optional) Triangle Rasterization: Functions to fill triangles (e.g., using scanline algorithms or barycentric coordinates).
- (Optional) Wireframe Rendering: Capability to draw the edges of 3D objects defined by vertices and edges/faces.
4. Basic Rendering Pipeline (Conceptual)
- (Optional) Vertex Transformation: Applying model-view-projection matrices to transform 3D object vertices into 2D screen coordinates.
- (Optional) Clipping: Basic logic to handle primitives partially or fully outside the screen boundaries.
Challenges and Design Choices
1. Handling Framebuffer Variations
- Problem: Linux framebuffers can have diverse pixel formats (bit depths, color order), strides (bytes per scanline), and memory layouts.
- Approach: Implemented logic to query framebuffer parameters via
ioctl
calls and adapt the pixel plotting function accordingly, potentially including color conversion routines.
2. Performance in C
- Problem: Drawing pixels or lines in tight loops can be computationally intensive. Direct memory access requires careful pointer arithmetic.
- Approach: Focused on using efficient algorithms (e.g., Bresenham for lines), optimizing inner loops, and minimizing redundant calculations. Careful management of memory allocation and pointers.
3. Mathematical Correctness
- Problem: Ensuring the accuracy of linear algebra functions (especially matrix operations like inversion or complex transformations) is crucial.
- Approach: Extensive testing of the math library components, potentially comparing outputs against known values or established libraries for verification. Modular design to isolate math logic.
4. Building Graphics Primitives from Scratch
- Problem: Implementing algorithms like line drawing or triangle rasterization correctly and efficiently requires careful attention to detail (edge cases, integer arithmetic).
- Approach: Studied and implemented standard algorithms, focusing on correctness first, then optimization. Debugging often involved visual output inspection.
Results / Current Capabilities
- Successfully initializes and draws pixels directly to the Linux framebuffer.
- Can render basic 2D primitives like lines and potentially triangles/polygons (wireframe or filled).
- Implements core 2D/3D vector and matrix operations.
- Applies basic transformations (translation, rotation, scaling) to rendered objects
Future Work
1. Advanced Rendering Features
- Implement solid triangle rasterization with Z-buffering for correct depth sorting in 3D scenes.
- Add basic lighting models (e.g., ambient, diffuse, specular; Gouraud or Phong shading).
- Explore texture mapping capabilities.
- Implement more sophisticated clipping algorithms (e.g., Sutherland-Hodgman).
2. Performance Optimization
- Further optimize drawing routines (e.g., assembly intrinsics, better caching strategies).
- Explore multi-threading for tasks like rasterization.
- Better parallelization and efficient computations need to be implemented
3. Input Handling
- Integrate basic input handling by reading from Linux input devices (
/dev/input/event*
) for interactive demos.
4. API Refinement
- Develop a cleaner, more user-friendly API for the library.
- Add more comprehensive error handling and documentation.
Conclusion
This project serves as a practical exploration into the foundational layers of computer graphics. By implementing a linear algebra library and rendering pipeline targeting the Linux framebuffer directly in C, it provides invaluable insights into low-level system interaction and the mathematics that power visual displays. It stands as a testament to building complex functionality from basic principles and offers a solid base for further graphics programming experimentation.
Repository and Resources
Code
The complete source code can be found here. The repository includes:
- The C source files for the linear algebra library.
- Framebuffer interface code.
- Rendering primitive implementations.
- A
Makefile
for building the project. - Example/demo programs.
Dependencies
- A C Compiler (GCC or Clang)
- Make (build utility)
- Linux Kernel with Framebuffer support enabled (e.g.,
CONFIG_FB
,CONFIG_FB_VESA
, or specific hardware drivers)
For detailed setup and compilation instructions, refer to the README.md
file in the repository.